本文给大家分享一下C#操作(读取、写入)XML文档的实用方法,即用.NET本身提供的Deserialize和Serialize进行反序列化和序列化XML文档。这种方法主要是对比较规范的XML文档进行操作,因为它(XML文档)和类对象是严格对应的,否则在反序列或序列化的时候会出现错误。其他的不用多说,直接看示例代码吧:
生成的XML文档:
using System;
using System.Collections.Generic;
using System.IO;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
using System.Xml.Serialization;
namespace XmlDemo
{
public class Program
{
private static readonly string _xmlPath = @"D:books.xml";
private static readonly string _xmlSinglePath = @"D:book.xml";
static void Main(string[] args)
{
Console.WriteLine("请按任意键写入XML文件[Single]...");
Console.ReadKey();
WriteSingleXml();
Console.WriteLine("请按任意键读取XML文件[Single]...");
Console.ReadKey();
ReadSingleXml();
Console.WriteLine("请按任意键写XML文件[List]...");
Console.ReadKey();
WriteListXml();
Console.WriteLine("请按任意键读取XML文件[List]...");
Console.ReadKey();
ReadListXml();
Console.ReadKey();
}
static void ReadSingleXml()
{
Book book;
var reader = new XmlSerializer(typeof(Book));
using (var fs = File.OpenRead(_xmlSinglePath))
{
book = reader.Deserialize(fs) as Book;
}
Console.WriteLine("Id:{0}", book.Id);
Console.WriteLine("Name:{0}", book.Name);
Console.WriteLine("Author:{0}", book.Author);
Console.WriteLine("Price:{0}", book.Price);
Console.WriteLine("---------------------------------------");
}
static void WriteSingleXml()
{
Book book = new Book { Id = 1, Author = "Lampo", Name = "C# Design pattern", Price = 55.8D };
var reader = new XmlSerializer(typeof(Book));
using (var fs = File.OpenWrite(_xmlSinglePath))
{
reader.Serialize(fs, book);
}
}
static void ReadListXml()
{
List<Book> books;
var reader = new XmlSerializer(typeof(List<Book>));
using (var fs = File.OpenRead(_xmlPath))
{
books = reader.Deserialize(fs) as List<Book>;
}
books.ForEach(x =>
{
Console.WriteLine("Id:{0}", x.Id);
Console.WriteLine("Name:{0}", x.Name);
Console.WriteLine("Author:{0}", x.Author);
Console.WriteLine("Price:{0}", x.Price);
Console.WriteLine("---------------------------------------");
});
}
static void WriteListXml()
{
List<Book> books = new List<Book>();
for (int i = 1; i < 5; i++)
{
books.Add(new Book { Id = i, Author = "Author " + i, Name = "Name " + i, Price = i * 5.0 });
}
var reader = new XmlSerializer(typeof(List<Book>));
using (var fs = File.OpenWrite(_xmlPath))
{
reader.Serialize(fs, books);
}
}
}
[Serializable]
public class Book
{
public int Id { get; set; }
public string Name { get; set; }
public string Author { get; set; }
public double Price { get; set; }
}
}
运行结果如图:
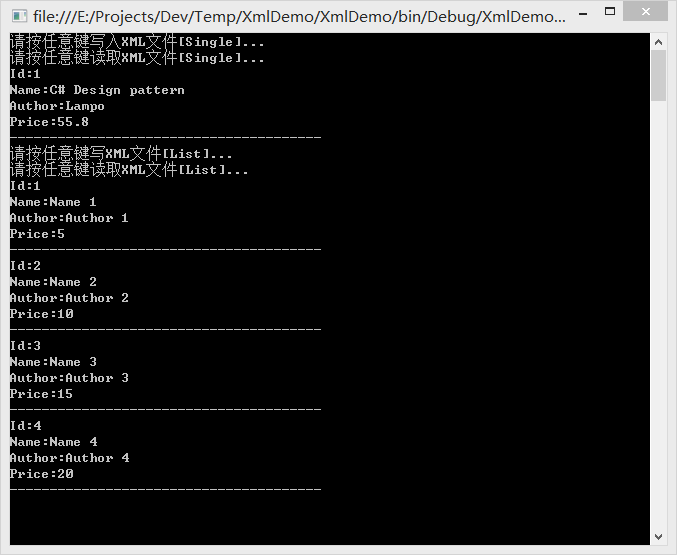
<?xml version="1.0"?>
<Book xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xmlns:xsd="http://www.w3.org/2001/XMLSchema">
<Id>1</Id>
<Name>C# Design pattern</Name>
<Author>Lampo</Author>
<Price>55.8</Price>
</Book>
版权声明:本作品系原创,版权归码友网所有,如未经许可,禁止任何形式转载,违者必究。
发表评论
登录用户才能发表评论, 请 登 录 或者 注册